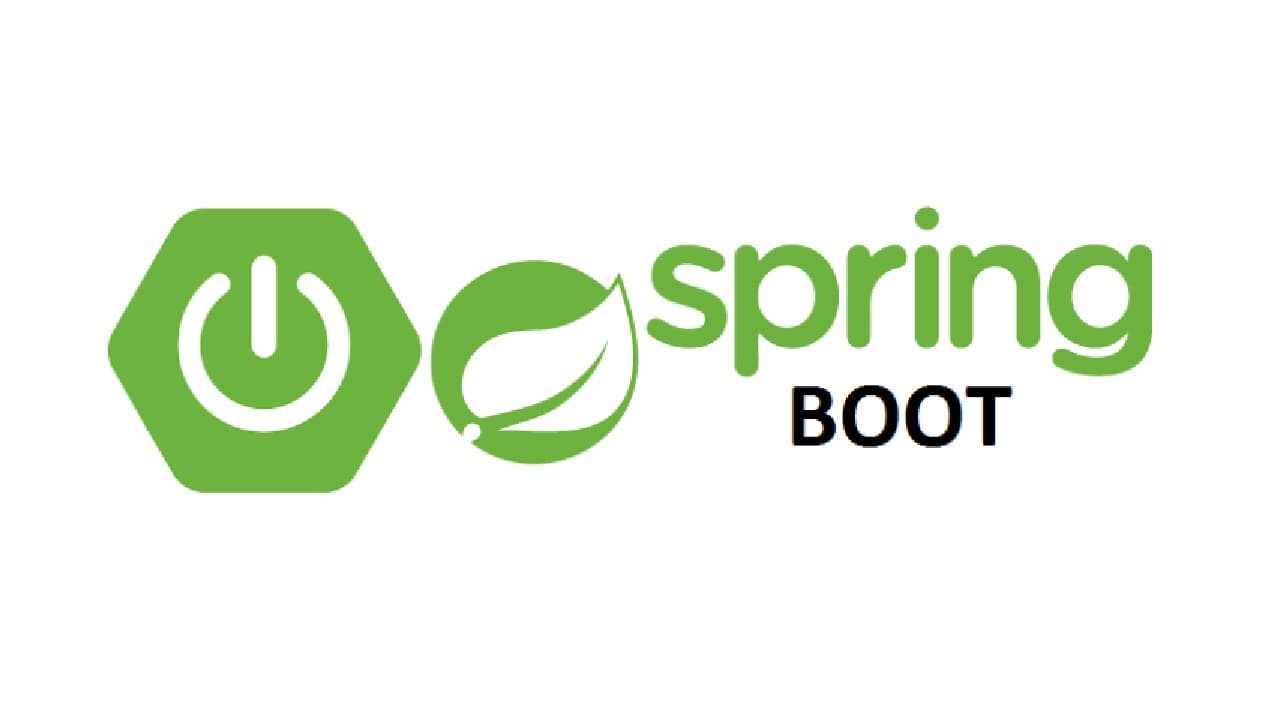
Spring Boot: Simplifying Java Application Development
A comprehensive guide to Spring Boot, its features, benefits, and practical code examples to help developers get started with creating Java-based applications effortlessly.
admin
@administrator
Introduction
Spring Boot is a Java-based framework that simplifies the development of Spring applications by providing default configurations, embedded servers, and pre-built components. It is widely used for building microservices, REST APIs, and enterprise-level applications.
Why Use Spring Boot?
- Auto-Configuration: Reduces the need for manual configurations.
- Embedded Server: No need to deploy to an external server; comes with embedded Tomcat, Jetty, or Undertow.
- Production-Ready Features: Includes monitoring, health checks, and metrics.
- Microservices-Friendly: Ideal for creating lightweight, decoupled services.
Key Features of Spring Boot
- Starter Dependencies: Simplifies dependency management.
- Spring Initializr: Quickly bootstrap a Spring project.
- Actuator: Adds production-ready monitoring.
- DevTools: Enhances the development experience with hot reload and debugging tools.
Getting Started with Spring Boot
1. Create a Spring Boot Project
The easiest way to start a Spring Boot application is through Spring Initializr.
2. Add Dependencies
Here’s an example of a pom.xml
for a Spring Boot application:
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> </dependency> </dependencies>
3. Create a REST API
Here’s how to build a simple REST API using Spring Boot:
Main Application Class
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
Controller
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController @RequestMapping("/api") public class HelloWorldController { @GetMapping("/hello") public String sayHello() { return "Hello, World!"; } }
4. Connect to a Database
application.properties
spring.datasource.url=jdbc:h2:mem:testdb spring.datasource.driverClassName=org.h2.Driver spring.datasource.username=sa spring.datasource.password= spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
JPA Entity
import jakarta.persistence.Entity; import jakarta.persistence.GeneratedValue; import jakarta.persistence.GenerationType; import jakarta.persistence.Id; @Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; private String email; // Getters and Setters }
Repository
import org.springframework.data.jpa.repository.JpaRepository; public interface UserRepository extends JpaRepository<User, Long> { }
Service
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.List; @Service public class UserService { @Autowired private UserRepository userRepository; public List<User> getAllUsers() { return userRepository.findAll(); } }
Controller
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import java.util.List; @RestController @RequestMapping("/api/users") public class UserController { @Autowired private UserService userService; @GetMapping public List<User> getUsers() { return userService.getAllUsers(); } }
5. Run the Application
Run the application using:
mvn spring-boot:run
Visit http://localhost:8080/api/hello
to see your REST API in action!
Advanced Features of Spring Boot
1. Spring Boot Actuator
Add Actuator dependency to your project:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency>
Actuator provides endpoints like:
/actuator/health
/actuator/metrics
2. Custom Exception Handling
import org.springframework.http.HttpStatus; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.bind.annotation.ResponseStatus; import org.springframework.web.bind.annotation.RestControllerAdvice; @RestControllerAdvice public class GlobalExceptionHandler { @ExceptionHandler(RuntimeException.class) @ResponseStatus(HttpStatus.BAD_REQUEST) public String handleRuntimeException(RuntimeException ex) { return ex.getMessage(); } }
3. Scheduling Tasks
import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Component; @Component public class TaskScheduler { @Scheduled(fixedRate = 5000) public void scheduledTask() { System.out.println("Task executed at: " + System.currentTimeMillis()); } }
Enable scheduling by adding @EnableScheduling
to your main class.
Conclusion
Spring Boot is an incredibly versatile and developer-friendly framework for creating robust Java applications. From its auto-configuration capabilities to its seamless integration with Spring modules, Spring Boot empowers developers to focus on building features rather than boilerplate code.
With its rich ecosystem and strong community support, Spring Boot continues to be a top choice for Java developers worldwide.
Have you tried building an application with Spring Boot? Share your experience in the comments below!